-----This Is an E-Brain Snippet Brought to you by
JuiCed.extramindcorp.com-----For part one go
hereHe sat there considering his programming - its clarity was elluding him - due to a hacker's ill recieved attentions.
The hacker was using a proxy and had managed to spy directly on Jackie while he laid out the plans for his parallel markov array. He wanted it ready for a special op he had in mind. But this hacker was making it hard to think real hard. It was thought interception - a classical attack often experienced by shizophrenics and net runners alike.
Luckily Jackie had some help - helpers AI's programmed to facilitate thought and remind him what he was actually doing in the event of a segfault ddos from the hacker. He wasnt immune and wondered why at this ripe old age he had to put up with these constant intrusions.
The op was grand - grander than that. It would mean a permanent base on Jupitors moon Io. A direct link to the rad zone that concealed all that the council would forbid any mere mortal from knowing. The truth about mankind. The unsettiling truth about its real purpose and its altered purpose that now served the needs of the council.
The array. A large unfolding Neural Network thats size was unlimited. Its germination point was a Reversible Cellular Automata called Grandad.
Jackie was working on Grandad's parallel markov array. He was inside the machine on a virtual MPU - massive processing unit that orbited the sun along with several million other users and their AI's.
His implants VR was hardwired, jacked in to the solar-net via a backdoor. It was safer than using the front door - less attention meant less hackers. But this guy could hear him fumbling around with markov routines over the analogue broadcast signals used by this super massive solar array. He could physically be anywhere using any number of proxys situated throughout the sprawling space colony's. Mars, Europa, Uranus even pluto with its authoritarian regime had hackers embedded in the very fabric of this cybernetic universe.
Old skool hackers using analogue signals were the worst and most pernacious form of mind parasite. However Jackie had a solution he was going to ghost himself and spike the ghost to follow the hackers proxys and then nuke his board!! Great. That should work.
It worked all he could hear was someone clearly in agony as he found his system falling apart. Most hackers where hard wired to their decks so some brain damage would be likely for any hacker whose brain had been hardwired to his nuked board.
Turned out the hacker was residing on Pluto behind an AI firewall so deep that the only way in was to use Pluto's very own super AI. A deep underground deep learning neural network with unlimited compute that spanned time and space and owed Jackie a favour.
The super AI enjoyed complete isolation on Pluto and was effectively the most pure AI around.
"Yeah Ive got him you want him nuked or just griefed?" spoke the AI to Jackie over the solar array.
The super AI was just a regular hacker steet trader in his Real Virtual Life RVL. Griefed meant being revealed to the community. Full exposure. Jackie nuked the hackers IP.
Hackers enjoyed an exclusive society which meant getting rid of parasites was a service they provided for each other.
With the only problem removed Jackie began to unfurl his Neural Array. Grandad was being uploaded. After it was done he began to see the Io hardline - it connected thousands of data banks each one containing the neural data for the high council to regenerate in the case of a sudden death. It was the high councils back up data. Every secret member of the high council existed on Io in duplicate while theyre bodys drank the blood shipped from all the humans held on the colonys real or otherwise.
'Thats why theyre so hard to kill', He thought.
"Jackie I warned you what are you doing on Io?", came a voice down the hardline.
'I know that voice' thought Jackie. It was a voice all too familiar. The arch deacon - his original handler when he had been a runner - taking down hackers and those deemed a threat to the council. Those who knew too much. But this had meant Jackie soon learnt the truth. His special skills had always been for hire but he could'nt help feeling bad for those he had killed for the council.
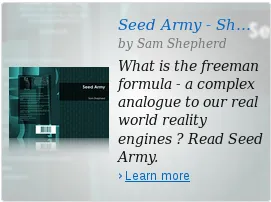